Recon (Reconnaissance) – The act of gathering important information on a target system. This information can be used to better attack the target. For example, open source search engines can be used to find data that can be used in a social engineering attack as well as set of custom tools for active steps of the
To build own automated recon tool in this article we going to merge several tools and pack them with web interface based on PHP for recon activities.
For what purpose do we need such tool? First to identify correct url to target web application with usage of domain name, get response headers and other debug information, identify firewall solution and used technologies stack of the application. Additionally our tool will be able to see WHOIS data of the domain owner.
Requirements
We going to use php version 7 (the same can be done with php5) with Apache, Linux distribution (debian) and several recon tools:
- cURL
- wafw00f
- Whois
- WhatWeb
In my case everything works on Kali Linux distribution, but it is not necessary to work only with it, you may use other linux distros as well like Ubuntu or Debian.
Installation
- How to install wafw00f
- $ apt-get install whois (to install whois tools on your system)
- Installing WhatWeb (see GitHub for installation process)
- cURL should be in your system by default
In Kali Linux you may find all this tools pre-installed right after you unpacked distribution and installed it on your machine.
Automated recon tool script
First we need to create 1 folder and 1 file:
$ mkdir /var/www/tmp
$ chmod 777 /var/www/tmp
We need this folder to keep our temporary XML files from WhatWeb output.
Now let’s create our php file with script:
$ touch /var/www/index.php
$ chmod 755 /var/www/index.php
On the following step we going to write some code (or you may just copy & paste code below) in our file “index.php”:
<?php
set_time_limit(0);
//ini_set('display_errors', 1);
//ini_set('display_startup_errors', 1);
//error_reporting(E_ALL);
function progress($item) {
echo $item;
ob_flush();
flush();
}
function url($target) {
$find_url = shell_exec("curl -I -L $target | awk '/Location/{print $2}'");
$res = explode("\n", trim($find_url));
$url = end($res);
if($url) {
$result = $url;
} else {
$result = "http://".$target;
}
return $result;
}
function whois($target) {
$whois = shell_exec("whois $target");
return $whois;
}
function firewall($url) {
$check_firewall = shell_exec("/usr/bin/wafw00f $url");
preg_match("/is\sbehind\sa\s(.+?)\n/", $check_firewall, $result);
if($result[1]) {
$firewall = $result[1];
} else {
$firewall = 'None';
}
return $firewall;
}
function debug($url) {
$debug = shell_exec("curl -I $url");
return $debug;
}
function whatweb($url) {
$check_stack = shell_exec("whatweb --no-errors $url --log-xml=/var/www/tmp/temp.xml");
$filepath = "/var/www/tmp/temp.xml";
$content = utf8_encode(file_get_contents($filepath));
$xml = simplexml_load_string($content, 'SimpleXMLElement', LIBXML_COMPACT | LIBXML_PARSEHUGE);
foreach($xml->target as $target) {
$array = array();
foreach($target->plugin as $key => $data) {
$array[] = array(
$key => $data
);
}
$res[] = array(
'target' => $target->uri,
'data' => $array
);
} $result = $res; unlink($filepath); return $result;
}
$target = trim($_POST['target']);
$submit = $_POST['submit'];
?>
Full script you can find at GitHub in my repository. Pay attention that my script from github is a bit different, so please don’t forget to checks paths and update them regarding your needs.
Now we going to walk through the used functions:
- url function – it used to identify proper url for specific domain. We checking with help of cURL for possible redirections and trying to identify valid (last).
- whois function – simply runs whois tool to get information about domain name owner. It uses linux package and executes it through php shell_exec functions.
- firewall functions – with help of “wafw00f” firewall identification script it may help your properly find out firewall solution used by the target system.
- function debug – this is just usage of linux cURL to get debug information from the server response.
- whatweb function – the most interesting one, as it helps us to understand technologies stack used on the target server / application. It can identify not only vendor type but also it’s version which can be helpful to find out vulnerabilities for outdated software.
How it works
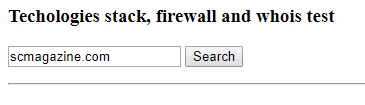
After we launch this script it will require to wait a bit for the results. In this script I wrote “progress” function to be able see results as soon as they are available with help of php “ob_flush” function. Below we can see the results:
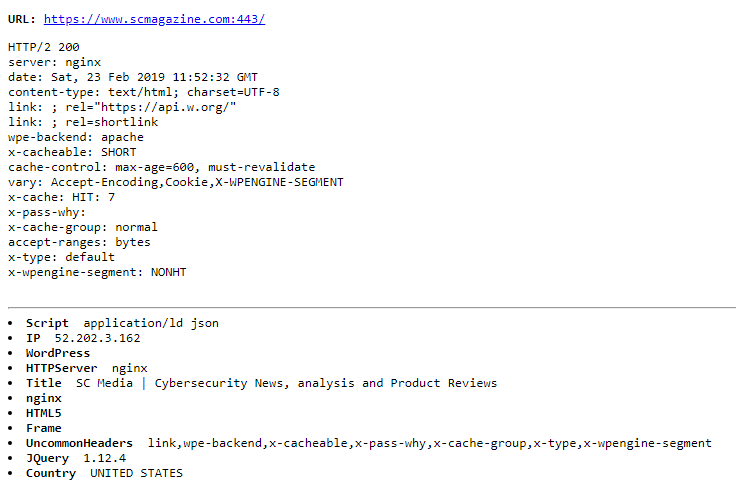
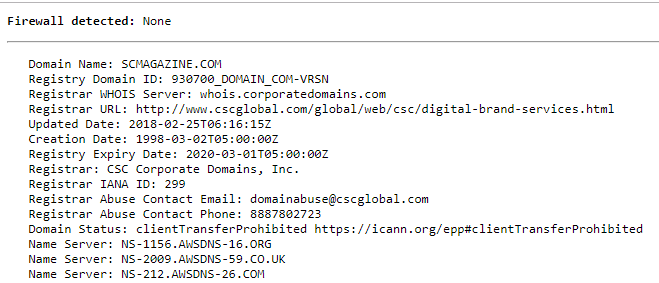
As we can see – there were no firewalls detected, though it was possible partly identify used stack and get some information from whois.
Now we can use several tools from one place which makes our future vulnerability assessments and penetration tests more easier on the recon phase.